Here is my full code:
function read_data() {
var workcenters = [];
var requestUriWorkCenters = "<URL_HIDDEN>/WorkcenterSet";
OData.read(requestUriWorkCenters, function(data, request) {
for (var i = 0; i < data.results.length; i++) {
workcenters.push({
key : data.results[i].WorkcenterId,
label : data.results[i].WorkcenterName
});
}
}, function(error) {
}
)
var nodes = [];
var hierarchy = [];
var requestUriOrders = "<URL_HIDDEN>OrderSet";
OData
.read(
requestUriOrders,
function(data, request) {
for (var i = 0; i < data.results.length; i++) {
nodes.push({
key : data.results[i].OrderId,
start_date : data.results[i].Start,
end_date : data.results[i].End,
text : data.results[i].OrderText,
workcenter_id : data.results[i].WorkcenterId
});
hierarchy.push({
key : data.results[i].OrderId,
label : data.results[i].OrderText,
open : true,
children : []
});
for (var j = 0; j < data.results[i].OrderToOperations.results.length; j++) {
nodes
.push({
key : data.results[i].OrderId
+ data.results[i].OrderToOperations.results[j].OperationId,
start_date : data.results[i].OrderToOperations.results[j].Start,
end_date : data.results[i].OrderToOperations.results[j].End,
text : data.results[i].OrderToOperations.results[j].OperationText,
workcenter_id : data.results[i].OrderToOperations.results[j].WorkcenterId
});
hierarchy[i].children
.push({
key : data.results[i].OrderId
+ data.results[i].OrderToOperations.results[j].OperationId,
label : data.results[i].OrderToOperations.results[j].OperationId
});
}
}
}, function(error) {
}
);
return [ nodes, hierarchy, workcenters ];
}
function init() {
var graphData = read_data();
var nodes = graphData[0];
var hierarchy = graphData[1];
var workcenters = graphData[2];
scheduler.locale.labels.timeline_tab = "Orders";
scheduler.locale.labels.newview_tab = "Resources";
scheduler.locale.labels.section_custom = "Section";
scheduler.locale.labels.unit_tab = "Unit";
scheduler.config.details_on_create = true;
scheduler.config.details_on_dblclick = true;
scheduler.config.xml_date = "%Y-%m-%d %H:%i";
scheduler.config.multisection = true;
scheduler.templates.event_class = function(start, end, event) {
var original = scheduler.getEvent(event.id);
if (!scheduler.isMultisectionEvent(original))
return "";
return "multisection workcenter_" + event.section_id;
};
// ===============
// Configuration
// ===============
scheduler.createTimelineView({
section_autoheight : false,
name : "timeline",
x_unit : "minute",
x_date : "%H:%i",
x_step : 30,
x_size : 24,
x_start : 16,
x_length : 48,
y_unit : hierarchy,
y_property : "key",
render : "tree",
folder_dy : 20,
dy : 60
});
scheduler.createTimelineView({
name : "resourceview",
x_unit : "minute",
x_date : "%H:%i",
x_step : 30,
x_size : 24,
x_start : 16,
x_length : 48,
y_unit : workcenters,
y_property : "workcenter_id",
render : "bar"
});
// ===============
// Data loading
// ===============
scheduler.config.lightbox.sections = [ {
name : "description",
height : 130,
map_to : "text",
type : "textarea",
focus : true
}, {
name : "custom",
height : 23,
type : "timeline",
options : null,
map_to : "workcenter_id"
}, // type should be the same as name of the tab
{
name : "time",
height : 72,
type : "time",
map_to : "auto"
} ]
scheduler.createUnitsView({
name : "unit",
property : "workcenter_id",
list : workcenters
});
scheduler.init('scheduler_here', new Date(2016, 10, 15), "day");
scheduler.parse(nodes, "json");
}
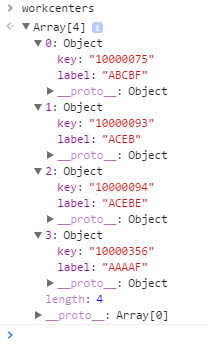
The “Resource” view and “Unit” view displays the Y and X axis as per the data, but no event data.
The “TimeLine” view does not display anything in the tree.