I’ve been trying for a day now to get the gantt chart working with Codeigniter. The folks at DHTMLX were nice enough to send me an example but their example used SqlLite and not MySQL so it didn’t quite work. I’ve made various changes to it but it still doesn’t work. Here’s my controller and view.
[code]<?php if ( ! defined(‘BASEPATH’)) exit(‘No direct script access allowed’);
require_once("./dhtmlx/connector/gantt_connector.php");
require_once("./dhtmlx/connector/db_phpci.php");
DataProcessor::$action_param =“dhx_editor_status”;
class Gantt extends CI_Controller {
public function index()
{
$this->load->view('gantt');
}
public function data()
{
$this->load->database();
//data feed
$gantt = new JSONGanttConnector($this->db, "PHPCI");
$gantt->mix("open", 1);
//$gantt->enable_order("sortorder");
$gantt->render_links("gantt_links", "id", "source,target,type");
$gantt->render_table("gantt_tasks","id","text,start_date,duration,progress,sortorder,parent","");
}
}
[/code]
And view
[code]
Basic initialization
html, body{ height:100%; padding:0px; margin:0px; overflow: hidden;}
gantt.config.xml_date = "%Y-%m-%d %H:%i:%s";
gantt.init("gantt_here");
gantt.load("<?=base_url();?>gantt/data");
var dp = new dataProcessor("<?=base_url();?>gantt/data");
dp.init(gantt);
dp.action_param = "dhx_editor_status";
</script>
[/code]
I would prefer to use a Model too but I’m not sure what it should look like. Can anyone help? I would be happy to provide my whole CI structure if anyone wants to see it.
Thanks if you can help.
Normally the DB type must not matter, as the connector’s code doesn’t work with DB directly. Please be sure that DB was correctly configured ( $this->db contains valid DB object in the above code ) and that DB has all necessary tables ( sql structure can be taken from samples\common\dump.sql )
As for models - unfortunately only one model object can be used with connector, and Gantt require two models ( first for tasks, second for links ).
Okay I’ll take a look at that.
Are there any examples of talking to the object without having to use the connector? In other words can I load with a JSON object from my controller and then save/update using AJAX/Jquery?
Yep, sure
Gantt can load data from json feed, so just output the json data by php script and use url of such action on client side, as parameter of load command.
DataSaving a bit more complicated, but can be done as well.
Each time when something changed in the gantt, it sends data to php script ( url set as parameter of dataProcessor constructor ) in the next way
docs.dhtmlx.com/doku.php?id=dhtm … gprinciple
So I have continued to try and make this control use the Connector but even if I just take the Scheduler which works just fine in my Codeigniter example and modify it to look just like the example I was emailed it still doesn’t load the data into the control.
It renders the control perfectly and I’ve even modified it to allow me to send the data and links to it from my controller and it adds the data when I use that method but it still doesn’t add the data if I try to use the DataConnector.
I suspect I must not have the required files in the required places and so it’s not loading something. I have used Chrome’s Inspector to look at the javascript and I had to modify a bit to make it load everything but I think there must be some folder or files I haven’t added to my folder structure.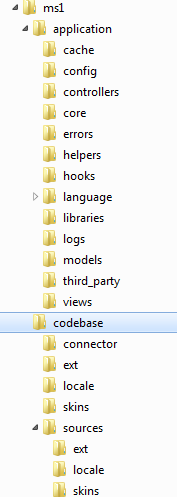
What am I missing?
I’ve been able to debug this and find out that the line that isn’t finishing is $gantt->render_table"gantt_tasks",“id”,“text,start_date,duration,progress,parent”,"");
The render_links line finishes but not the render_table.
Perhaps that tells you something more?
You can grab the latest connector from here
github.com/DHTMLX/connector-php
On client side you need to have only dhtmlxscheduler.js, there is no need for any other js files.
In case of connector you can enable logging, that can provide some useful output.
docs.dhtmlx.com/doku.php?id=dhtm … tor:errors
I’m working with the Gannt chart so I don’t understand if you really mean I need the dhtmlxscheduler.js or if you misread my post and you really meant I need the dhtmlxgantt.js.
I will turn on logging but I found that the render_table statement is ending due to hitting the end_run function. Is it normal to end using that or should it never hit that during normal operation?
Sorry, was a my typo, of course it must be dhtmlxgantt.js
render_table statement is ending due to hitting the end_run function
render_table ends the current script execution. This command triggers data loading or data saving, generates and outputs response xml|json. There is no point to further script processing. It is by design.
I’m not sure if I’m actually getting anywhere but here’s what I’ve seen. In the first 2 cases I have the gantt.load section saying xml as the second parameter.
If I use localhost the data kind of loads but it throws an error with a bunch of modal boxes that all say “Invalid day index”. It also only loads some data into the tree view side and doesn’t load anything into the graph side. The data on the tree view side is also just numbers instead of the text and all durations are 0.
If I use the actual IP of the machine I get a popup saying “Error Type: Improper XML” and something else on the popup about “Description: Incorrect XML”.
If I change the second parameter to json then I don’t get any data loaded either way but if I use the IP I get a modal that says “Invalid data”.
This is really getting me annoyed. I really prefer this control to anything else I’ve seen and I would like to purchase it but I’m not going to do that until I can make it work.
If I use the actual IP of the machine I get a popup saying “Error Type: Improper XML” and something else on the popup about “Description: Incorrect XML”.
This one is most probably caused by the cross-domain security
if you are using full paths, be sure that domain of html page and domain in data url are the same.
If you don’t think that problem is domain related - try to load the data url directly in a browser. It may show some details about error.
As for json, to work with json be sure that both client and server side are correctly configured. You need to use JsonGanttConnector on server side and load(url, “json”) on client side.
I was getting the same “Invalid Day Index”. I figured it out because I had a lot of durations=0. This simply meant that the task started and ended that same day. I changed it by simply noting that it was a 1 instead of a 0.
I have also integrated dhtmlxGantt with Yii and MySQL. It was a bit tedious to get the JSON format that was needed, but it works beautifully.
After weeks of working on this off and on I finally have it working with Codeigniter.
Now I need to modify the editor to add a few more fields/text boxes.
It’s a little unclear in the documentation as to how to deal with saving and loading extra elements from the database but it looks like I need to use the map_to function.
So I believe I create a new field in my database and add that field to the list of fields in my controller and I think it will work.
I’m not sure if I add it to the second set of fields in the list like such:
$gantt->render_table(“gantt_tasks”,“id”,“start_date,duration,text,parent,progress,extra_element”,"");
or if it goes in the third set like such:
$gantt->render_table(“gantt_tasks”,“id”,“start_date,duration,text,parent,progress”,“extra_element”);
Of course I’ll modify my code and see if I can get it working using one of those options but figured I would put this here in case someone sees it and chimes in.
Looks like I’ll be having my purchasing guy buy a copy shortly. 
Just add it to the existing list of the fields
$gantt->render_table("gantt_tasks","id","start_date,duration,text,parent,progress,extra_element","");
all fields from this list will be available on the client side. And you can use “map” in configuration of lightbox to map html inputs to event properties.